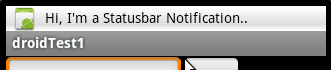
A status bar notification is used to notify the user of a system event, like an sms being received or a new device being detected, without interrupting the user from whatever other task they might be doing with their phone.
The status bar area is located at the top of the screen and the user can pull this area to expand it, and show a history of notifications.
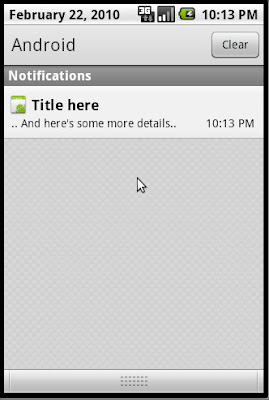
If a Notification has been setup to include an enclosed Intent, selecting that particular notification in this expanded view can fire the Intent, taking the user to an Activity screen of the related application, or do any of the many other things that Intents can do.
You can also configure the notification to alert the user with a sound, a vibration, and flashing lights on the device.
A background Service should never launch an Activity on its own in order to receive user interaction. The Service should instead create a status bar notification that will launch the Activity when selected by the user.
A status bar notification should be used for any case in which a background Service needs to alert the user about an event.
Here is a simple method that creates and displays a notification when passed a string
msg
: public void displayNotification(String msg)
{
NotificationManager manager = (NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE);
Notification notification = new Notification(R.drawable.icon, msg, System.currentTimeMillis());
// The PendingIntent will launch activity if the user selects this notification
PendingIntent contentIntent = PendingIntent.getActivity(this, REQUEST_CODE, new Intent(this, ExpandNotification.class), 0);
notification.setLatestEventInfo(this, "Title here", ".. And here's some more details..", contentIntent);
manager.notify(NOTIFICATION_ID, notification);
}
.. Which you can call simply like this:
displayNotification("Hi, I'm a Statusbar Notification..");
.. Easy, isn't it?
Hi,
ReplyDeletewhat are the NOTIFICATION_ID and REQUEST_CODE?
What values should they be set to?
Hi Great nintendoDS Lover,
ReplyDeleteFor this example, I used :
...
private static final int NOTIFICATION_ID=2;
private static final int REQUEST_CODE=1;
...
declare these fields just after your ..
extends Activity{..
.. These are just used to help you identify what notifications and requests are being triggered, so pretty much any integer will do.
Let me know how you go.
G
notification.flags=Notification.FLAG_AUTO_CANCEL;...?
ReplyDeletehi...how to write on click event for button in notification message....
ReplyDeletewhere is this code put? Where do you go to type/send new notifications.?
ReplyDeleteHi Dennis,
ReplyDelete.. This is just an example method and a call to it. You can put it anywhere in your Activity code.
Whenever you want to make a new statusbar notification, you can reference it with your version of the 'displayNotification("Hi, I'm a Statusbar Notification..");' code.
Does that make sense?
I'm not really sure if that answers your question.
If not, can you give me some more details?
Cheers,
Glenn
Hi,
ReplyDeleteIs there any mechanism that the Notification bar is automatically expanded using code, instead of the user pulling down the notification bar.
Like when we press menu key on home screen and select notifications, the area is automatically expanded, i wanted to implement the same when a button is clicked in my application.
Sudhaker
Hi Glen,
ReplyDeleteIn an earlier answer you say the following:
declare these fields just after your ..
extends Activity{..
but "extends Activity" doesn't appear in your code?
Use this Easy to use library,
ReplyDeletehttp://code.google.com/p/android-easy-library/
The library, allows you to create a notification very easily, To create a notification, just use the following code,
EasyNotification en1 = new EasyNotification(this,0);
en1.showNotification();
You can also mofify other parameters of the notification, all that is given in the documentation within it.
Hi,
ReplyDeleteWhat is the ExpandNotification.class used for ? Where does this class comes from ?
Thank
Sam
Hi Samous,
ReplyDeleteExpandNotification.class is shown above in the creation of a new intent.
This new intent would be triggered when the user selects the notification, so ExpandNotification.class can be any class extending Activity that you would like to be opened when the notification is selected.
Cheers,
Glenn
Simple and Best..
ReplyDeleteWhy can't http://developer.android.com provide clear easy to understand examples like yours? THANKS!
ReplyDelete